LLEMU C API module
LLEMU - Legacy Lcd EMUlator.
Contents
- Reference
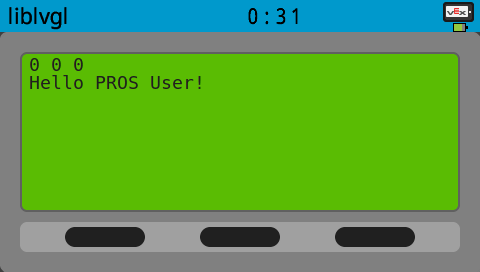
LLEMU provides a virtual 40x8 LCD screen with 3 buttons. The user can set the text of the screen and set create functions that are run when the buttons are pressed.
LLEMU is a emulation of the UART-based LCD screens that were available with VEX's cortex product line.
Files
- file llemu.h
Namespaces
- namespace pros::c
Functions
- bool lcd_is_initialized(void)
- Checks whether the emulated three-button LCD has already been initialized.
- bool lcd_initialize(void)
- Creates an emulation of the three-button, UART-based VEX LCD on the display.
- bool lcd_shutdown(void)
- Turns off the Legacy LCD Emulator.
- bool lcd_print(int16_t line, const char* fmt, ...)
- Displays a formatted string on the emulated three-button LCD screen.
- bool lcd_set_text(int16_t line, const char* text)
- Displays a string on the emulated three-button LCD screen.
- bool lcd_clear(void)
- Clears the contents of the emulated three-button LCD screen.
- bool lcd_clear_line(int16_t line)
- Clears the contents of a line of the emulated three-button LCD screen.
- bool lcd_register_btn0_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the leftmost button.
- bool lcd_register_btn1_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the center button.
- bool lcd_register_btn2_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the rightmost button.
- uint8_t lcd_read_buttons(void)
- Gets the button status from the emulated three-button LCD.
- void lcd_set_text_align(text_align_e_t alignment)
- Changes the alignment of text on the LCD background.
Enums
- enum lcd_text_align_e { LCD_TEXT_ALIGN_LEFT = 0, LCD_TEXT_ALIGN_CENTER = 1, LCD_TEXT_ALIGN_RIGHT = 2 }
- Represents how to align the text in the LCD.
Function documentation
bool lcd_is_initialized(void)
#include <liblvgl/include/liblvgl/llemu.h>
Checks whether the emulated three-button LCD has already been initialized.
Returns | True if the LCD has been initialized or false if not. |
---|
Example
if (pros::c::lcd_is_initialized()) { pros::c::lcd_print("LLEMU!"); } else { printf("Error: LLEMU is not initialized\n"); }
bool lcd_initialize(void)
#include <liblvgl/include/liblvgl/llemu.h>
Creates an emulation of the three-button, UART-based VEX LCD on the display.
Returns | True if the LCD was successfully initialized, or false if it has already been initialized. |
---|
Example
#include "pros/llemu.h" void initialize() { if (pros::c::lcd_initialize()) { pros::c::lcd_print("LLEMU!"); } else { printf("Error: LLEMU could not initailize\n"); } }
bool lcd_shutdown(void)
#include <liblvgl/include/liblvgl/llemu.h>
Turns off the Legacy LCD Emulator.
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
---|
Calling this function will clear the entire display, and you will not be able to call any further LLEMU functions until another call to lcd_initialize.
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void disabled() { pros::c::lcd_shutdown(); }
bool lcd_print(int16_t line,
const char* fmt,
...)
#include <liblvgl/include/liblvgl/llemu.h>
Displays a formatted string on the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line on which to display the text [0-7] |
fmt | Format string |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void initialize() { pros::c::lcd_initialize(); pros::c::lcd_print(0, "My formatted text: %d!", 2); }
bool lcd_set_text(int16_t line,
const char* text)
#include <liblvgl/include/liblvgl/llemu.h>
Displays a string on the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line on which to display the text [0-7] |
text | The text to display |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void initialize() { pros::c::lcd_initialize(); pros::c::lcd_set_text(0, "My custom LLEMU text!"); }
bool lcd_clear(void)
#include <liblvgl/include/liblvgl/llemu.h>
Clears the contents of the emulated three-button LCD screen.
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
---|
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void initialize() { pros::c::lcd_initialize(); pros::c::lcd_clear(); // Clear the LCD screen }
bool lcd_clear_line(int16_t line)
#include <liblvgl/include/liblvgl/llemu.h>
Clears the contents of a line of the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line to clear |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void initialize() { pros::c::lcd_initialize(); pros::c::lcd_clear_line(0); // Clear line 0 }
bool lcd_register_btn0_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.h>
Registers a callback function for the leftmost button.
Parameters | |
---|---|
cb | A callback function of type lcd_btn_cb_fn_t (void (*cb)(void)) |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
When the leftmost button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void left_callback() { static int i = 0; i++; pros::c::lcd_print(0, "Left button pressed %i times", i); } void initialize() { pros::c::lcd_initialize(); pros::c::lcd_register_btn0_cb(); }
bool lcd_register_btn1_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.h>
Registers a callback function for the center button.
Parameters | |
---|---|
cb | A callback function of type lcd_btn_cb_fn_t (void (*cb)(void)) |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
When the center button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void center_callback() { static int i = 0; i++; pros::c::lcd_print(0, "Center button pressed %i times", i); } void initialize() { pros::c::lcd_initialize(); pros::c::lcd_register_btn1_cb(); }
bool lcd_register_btn2_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.h>
Registers a callback function for the rightmost button.
Parameters | |
---|---|
cb | A callback function of type lcd_btn_cb_fn_t (void (*cb)(void)) |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
When the rightmost button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_
Example
#include "pros/llemu.h" void right_callback() { static int i = 0; i++; pros::c::lcd_print(0, "Right button pressed %i times", i); } void initialize() { pros::c::lcd_initialize(); pros::c::lcd_register_btn2_cb(); }
uint8_t lcd_read_buttons(void)
#include <liblvgl/include/liblvgl/llemu.h>
Gets the button status from the emulated three-button LCD.
Returns | The buttons pressed as a bit mask |
---|
The value returned is a 3-bit integer where 1 0 0 indicates the left button is pressed, 0 1 0 indicates the center button is pressed, and 0 0 1 indicates the right button is pressed. 0 is returned if no buttons are currently being pressed.
Note that this function is provided for legacy API compatibility purposes, with the caveat that the V5 touch screen does not actually support pressing multiple points on the screen at the same time.
void lcd_set_text_align(text_align_e_t alignment)
#include <liblvgl/include/liblvgl/llemu.h>
Changes the alignment of text on the LCD background.
Parameters | |
---|---|
alignment | An enum specifying the alignment. Available alignments are: TEXT_ALIGN_LEFT TEXT_ALIGN_RIGHT TEXT_ALIGN_CENTER |
Example
#include "pros/llemu.h" void initialize() { pros::c::lcd_initialize(); pros::c::lcd_set_alignment(pros::c::lcd_Text_Align::LEFT); pros::c::lcd_print(0, "Left Aligned Text"); pros::c::lcd_set_alignment(pros::c::lcd_Text_Align::CENTER); pros::c::lcd_print(1, "Center Aligned Text"); pros::c::lcd_set_alignment(pros::c::lcd_Text_Align::RIGHT); pros::c::lcd_print(2, "Right Aligned Text"); }
Enum documentation
enum lcd_text_align_e
#include <liblvgl/include/liblvgl/llemu.h>
Represents how to align the text in the LCD.
Enumerators | |
---|---|
LCD_TEXT_ALIGN_LEFT |
Align the text to the left side of LCD line. |
LCD_TEXT_ALIGN_CENTER |
Align the text to the center of the LCD line. |
LCD_TEXT_ALIGN_RIGHT |
Align the text to the right side of the LCD line. |