LLEMU C++ API module
LLEMU - Legacy Lcd EMUlator.
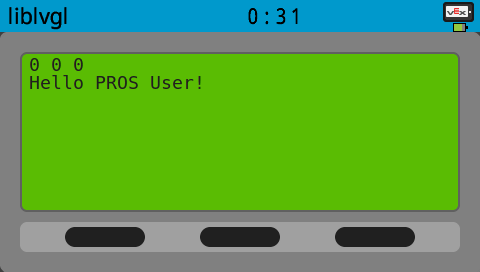
LLEMU provides a virtual 40x8 LCD screen with 3 buttons. The user can set the text of the screen and set create functions that are run when the buttons are pressed.
LLEMU is a emulation of the UART-based LCD screens that were available with VEX's cortex product line.
Files
Namespaces
- namespace pros::lcd
Functions
-
template<typename... Params>bool print(std::int16_t line, const char* fmt, Params... args)
- Displays a formatted string on the emulated three-button LCD screen.
- bool is_initialized(void)
- Checks whether the emulated three-button LCD has already been initialized.
- bool initialize(void)
- Creates an emulation of the three-button, UART-based VEX LCD on the display.
- bool shutdown(void)
- Turns off the Legacy LCD Emulator.
- bool set_text(std::int16_t line, std::string text)
- Displays a string on the emulated three-button LCD screen.
- bool clear(void)
- Clears the contents of the emulated three-button LCD screen.
- bool clear_line(std::int16_t line)
- Clears the contents of a line of the emulated three-button LCD screen.
- void register_btn0_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the leftmost button.
- void register_btn1_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the center button.
- void register_btn2_cb(lcd_btn_cb_fn_t cb)
- Registers a callback function for the rightmost button.
- void set_text_align(Text_Align alignment)
- Sets the alignment to use for subsequent calls that print text to a line.
- std::uint8_t read_buttons(void)
- Gets the button status from the emulated three-button LCD.
Enums
- enum class Text_Align { LEFT = 0, CENTER = 1, RIGHT = 2 }
- Represents how to align the text in the LCD.
Typedefs
- using lcd_btn_cb_fn_t = void(*)(void)
Defines
- #define LCD_BTN_LEFT
- #define LCD_BTN_CENTER
- #define LCD_BTN_RIGHT
Function documentation
#include <pros/llemu.hpp>
template<typename... Params>
bool print(std::int16_t line,
const char* fmt,
Params... args)
Displays a formatted string on the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line on which to display the text [0-7] |
fmt | Format string |
args | |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_initialize() first. EINVAL - The line number specified is not in the range [0-7]
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); pros::lcd::print(0, "My formatted text: %d!", 2); }
bool is_initialized(void)
#include <liblvgl/include/liblvgl/llemu.hpp>
Checks whether the emulated three-button LCD has already been initialized.
Returns | True if the LCD has been initialized or false if not. |
---|
Example
if (pros::lcd::is_initialized()) { pros::lcd::print("LLEMU!"); } else { printf("Error: LLEMU is not initialized\n"); }
bool initialize(void)
#include <liblvgl/include/liblvgl/llemu.hpp>
Creates an emulation of the three-button, UART-based VEX LCD on the display.
Returns | True if the LCD was successfully initialized, or false if it has already been initialized. |
---|
Example
#include "pros/llemu.hpp" void initialize() { if (pros::lcd::initialize()) { pros::lcd::print("LLEMU!"); } else { printf("Error: LLEMU could not initailize\n"); } }
bool shutdown(void)
#include <liblvgl/include/liblvgl/llemu.hpp>
Turns off the Legacy LCD Emulator.
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
---|
Calling this function will clear the entire display, and you will not be able to call any further LLEMU functions until another call to lcd_initialize.
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_initialize() first.
Example
#include "pros/llemu.hpp" void disabled() { pros::lcd::shutdown(); }
bool set_text(std::int16_t line,
std::string text)
#include <liblvgl/include/liblvgl/llemu.hpp>
Displays a string on the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line on which to display the text [0-7] |
text | The text to display |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_initialize() first. EINVAL - The line number specified is not in the range [0-7]
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); pros::lcd::set_text(0, "My custom LLEMU text!"); }
bool clear(void)
#include <liblvgl/include/liblvgl/llemu.hpp>
Clears the contents of the emulated three-button LCD screen.
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
---|
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_initialize() first. EINVAL - The line number specified is not in the range [0-7]
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); pros::lcd::clear(); // Clear the LCD screen }
bool clear_line(std::int16_t line)
#include <liblvgl/include/liblvgl/llemu.hpp>
Clears the contents of a line of the emulated three-button LCD screen.
Parameters | |
---|---|
line | The line to clear |
Returns | True if the operation was successful, or false otherwise, setting errno values as specified above. |
This function uses the following values of errno when an error state is reached: ENXIO - The LCD has not been initialized. Call lcd_initialize() first. EINVAL - The line number specified is not in the range [0-7]
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); pros::lcd::clear_line(0); // Clear line 0 }
void register_btn0_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.hpp>
Registers a callback function for the leftmost button.
Parameters | |
---|---|
cb | A callback function of type lcd_ |
When the leftmost button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
Example
#include "pros/llemu.hpp" void left_callback() { static int i = 0; pros::lcd::print(0, "Left button pressed %i times", i); i++ } void initialize() { pros::lcd::initialize(); pros::lcd::register_btn0_cb(); }
void register_btn1_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.hpp>
Registers a callback function for the center button.
Parameters | |
---|---|
cb | A callback function of type lcd_ |
When the center button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
Example
#include "pros/llemu.hpp" void center_callback() { static int i = 0; pros::lcd::print(0, "Center button pressed %i times", i); i++ } void initialize() { pros::lcd::initialize(); pros::lcd::register_btn1_cb(); }
void register_btn2_cb(lcd_btn_cb_fn_t cb)
#include <liblvgl/include/liblvgl/llemu.hpp>
Registers a callback function for the rightmost button.
Parameters | |
---|---|
cb | A callback function of type lcd_ |
When the rightmost button on the emulated three-button LCD is pressed, the user-provided callback function will be invoked.
Example
#include "pros/llemu.hpp" void right_callback() { static int i = 0; pros::lcd::print(0, "Right button pressed %i times", i); i++ } void initialize() { pros::lcd::initialize(); pros::lcd::register_btn2_cb(); }
void set_text_align(Text_Align alignment)
#include <liblvgl/include/liblvgl/llemu.hpp>
Sets the alignment to use for subsequent calls that print text to a line.
Parameters | |
---|---|
alignment | An enum specifying the alignment. Available alignments are: TEXT_ALIGN_LEFT TEXT_ALIGN_RIGHT TEXT_ALIGN_CENTER |
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); pros::lcd::set_alignment(pros::lcd::Text_Align::LEFT); pros::lcd::print(0, "Left Aligned Text"); pros::lcd::set_alignment(pros::lcd::Text_Align::CENTER); pros::lcd::print(1, "Center Aligned Text"); pros::lcd::set_alignment(pros::lcd::Text_Align::RIGHT); pros::lcd::print(2, "Right Aligned Text"); }
std::uint8_t read_buttons(void)
#include <liblvgl/include/liblvgl/llemu.hpp>
Gets the button status from the emulated three-button LCD.
Returns | The buttons pressed as a bit mask |
---|
The value returned is a 3-bit integer where 1 0 0 indicates the left button is pressed, 0 1 0 indicates the center button is pressed, and 0 0 1 indicates the right button is pressed. 0 is returned if no buttons are currently being pressed.
Note that this function is provided for legacy API compatibility purposes, with the caveat that the V5 touch screen does not actually support pressing multiple points on the screen at the same time.
Example
#include "pros/llemu.hpp" void initialize() { pros::lcd::initialize(); } void opcontrol() { while(true) { std::uint8_t state = pros::lcd::read_buttons(); pros::lcd::print(0, "%d %d %d", (state & LCD_BTN_LEFT) >> 2 (state & LCD_BTN_CENTER) >> 1, (state & LCD_BTN_RIGHT) >> 0 ); pros::delay(10); } }
Enum documentation
enum class Text_Align
#include <liblvgl/include/liblvgl/llemu.hpp>
Represents how to align the text in the LCD.
Enumerators | |
---|---|
LEFT |
Align the text to the left side of LCD line. |
CENTER |
Align the text to the center of the LCD line. |
RIGHT |
Align the text to the right side of the LCD line. |
Typedef documentation
typedef void(*)(void) lcd_btn_cb_fn_t
#include <pros/llemu.hpp>
Define documentation
#define LCD_BTN_LEFT
#include <pros/llemu.hpp>
#define LCD_BTN_CENTER
#include <pros/llemu.hpp>
#define LCD_BTN_RIGHT
#include <pros/llemu.hpp>